🌟Strings
Strings in Python are actually a sequence, so python can use indexes to call parts of the sequence. Indexing starts at 0 for python
Indexing – Extracting single character at particular index
Slicing – Subset of string
df.sort_values(by = ['Col_name'], ascending = False)
Built in String Methods
Some of the built in functions are like upper(), lower(), type()
For more information regarding built in functions, please refer to the link
Precedence of Operators in Python
The operator precedence in Python is listed in the following table. It is in descending order (upper group has higher precedence than the lower ones).
Operators | Meaning |
---|---|
() | Parentheses |
** | Exponent |
+x , -x , ~x | Unary plus, Unary minus, Bitwise NOT |
* , / , // , % | Multiplication, Division, Floor division, Modulus |
+ , - | Addition, Subtraction |
<< , >> | Bitwise shift operators |
& | Bitwise AND |
^ | Bitwise XOR |
| | Bitwise OR |
== , != , > , >= , < , <= , is , is not , in , not in | Comparisons, Identity, Membership operators |
not | Logical NOT |
and | Logical AND |
or | Logical OR |
Print Formatting
format() – Method to add formatted objects to printed string statements
For Eg:
name = "krishna"
age = 25
print(f"My name is {name} and my age is {age}")
print("My Name is %s and my age is %s" %(name,age))
print("My Name is {} and my age is {}".format(name,age))
🌟Numbers
Python has various “types” of numbers (numeric literals). We’ll mainly focus on integers and floating point numbers.
Variable Assignments
The names you use when creating these labels need to follow a few rules:
- Names can not start with a number.
- There can be no spaces in the name, use _ instead.
- Can’t use any of these symbols :'”,<>/?|()!@#$%^&*~-+
Data Types
Everything in Python is an “object”, including integers/floats
Most common and important types (classes)
– “Single value”: None, int, float, bool, str, complex
– “Multiple values”: list, tuple, set, dict
🌟Lists
Lists can be thought of the most general version of a sequence in Python.
Lists are
- Ordered : Maintain the order of data insertion
- Changeable : Lists are mutable and can modify items
- Heterogenous : List can contain data of different data types
- Allows duplicate data
a = [22, "krish", 7.0, ["a","b"]]
Just like strings, the len() function will tell you how many items are in the sequence of the list.
Add new element to the list : Append and Extend
Append function in Python adds a single element to the end of the list, whereas the extend function adds multiple elements (from an iterable) to the list.
list = [1,2,4]
list.append([5,6,7])
Output : [1,2,4,[5,6,7]]
list.extend([5,6,7])
Output : [1,2,4,5,6,7]
Slicing
The range()
function returns a sequence of numbers, starting from 0 by default, and increments by 1 (by default), and stops before a specified number.
Slicing is used to access individual elements or a rage of elements in a list.
Python supports “slicing” indexable sequences. The syntax for slicing lists is:
list_object[start:end:step]
orlist_object[start:end]
start and end are indices (start inclusive, end exclusive). All slicing values are optional.
.insert
: Theinsert()
method inserts the specified value at the specified position.
fruits = ['apple','banana','cherry']
fruits.insert(1, "orange")
Output : ['apple', 'orange', 'banana', 'cherry']
🌟Dictionaries (key value pair) 🚀
A Python dictionary consists of a key and then an associated value. That value can be almost any Python object.
Dictonaries are
- Un-Ordered : Items are stored with out index value
- Changeable : Dictonaries are mutable and can modify items
- Unique : Keys in Dict. should be unique
🗒️ A quick note, Python has a built-in method of doing a self subtraction or addition (or multiplication or division). We could have also used += or -= for the above statement.
🌟Sets
Sets are an unordered collection of unique elements. We can construct them by using the set() function.
.add() to add elements in set
.remove() to remove an element in the set
🌟Tuples
Tuples are used to store multiple items in a single variable.
Tuple is one of 4 built-in data types in Python used to store collections of data, the other 3 are List, Set, and Dictionary, all with different qualities and usage.
A tuple is a collection which is ordered and unchangeable.
Tuples are written with round brackets.
Summary of Data Types
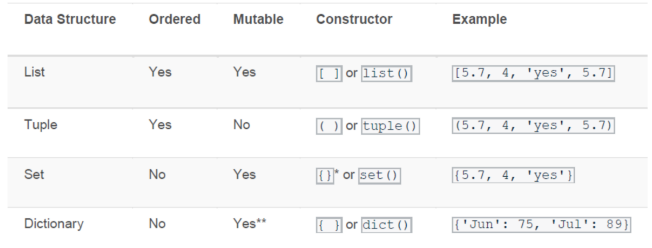
🌟Conditional Statements
If, else, elif
if Statements in Python allows us to tell the computer to perform alternative actions based on a certain set of results.
For Loop
A for loop acts as an iterator in Python, it goes through items that are in a sequence or any other iterable item. Objects that we’ve learned about that we can iterate over include strings,lists,tuples, and even built in iterables for dictionaries, such as the keys or values.
while Loop
The while statement in Python is one of most general ways to perform iteration. A while statement will repeatedly execute a single statement or group of statements as long as the condition is true. The reason it is called a ‘loop’ is because the code statements are looped through over and over again until the condition is no longer met.